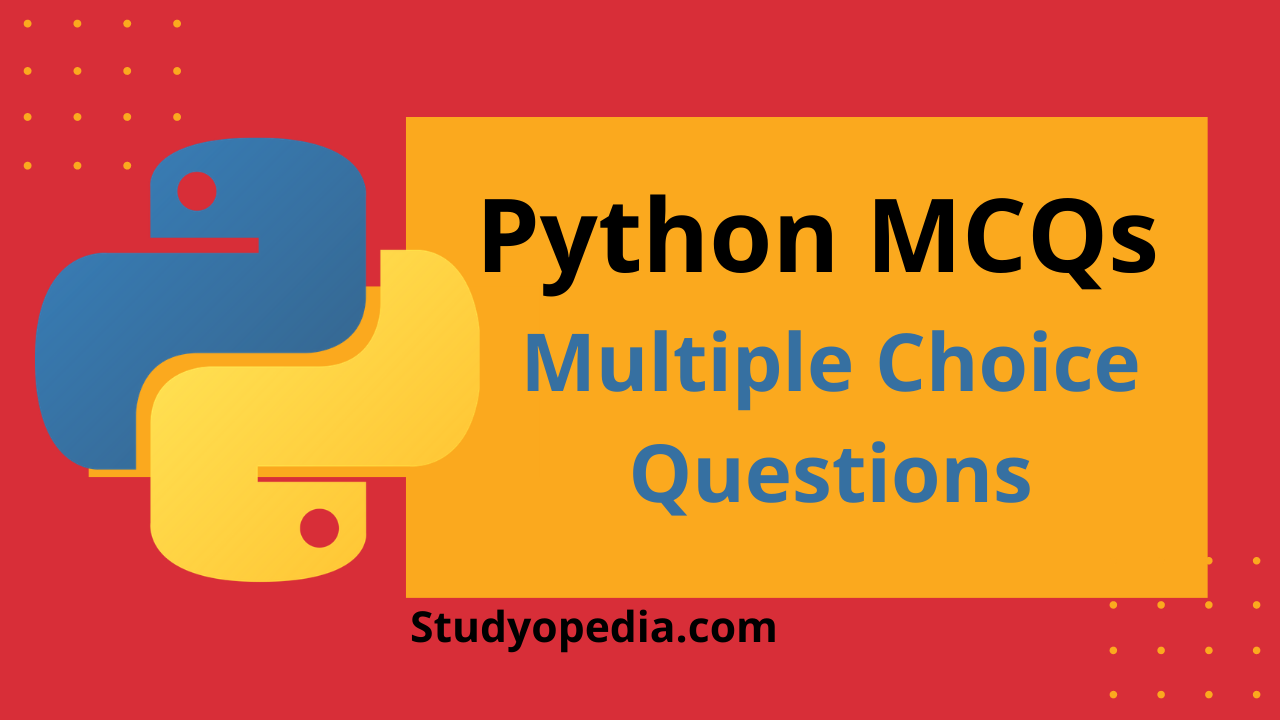
27 Jul Python Multiple Choice Questions (MCQs)
The Python Multiple Choice Questions and Answers are created from topics referenced around the web and the best-authored books. Polish your skills with these MCQs and assist yourself in attaining knowledge for Interviews, Entrance Examinations, Online Quizzes, etc. It is suggested to revise your Python concepts before beginning.
Note: Answers are marked in Bold
Top 70 Python Multiple-Choice Questions &Answers
Let’s begin!
1. Do we need to compile a program before execution in Python?
- Yes
- No
2. How to output the string “May the odds favor you” in Python?
- print(“May the odds favor you”)
- echo(“May the odds favor you”)
- System.out(“May the odds favor you”)
- printf(“May the odds favor you”)
3. How to create a variable in Python with a value 22.6?
- int a = 22.6
- a = 22.6
- Integer a = 22.6
- None of the above
4. How to add a single-line comment in Python?
- /* This is a comment */
- !! This is a comment
- // This is a comment
- # This is a comment
5. How to represent 261500000 as a floating number in Python?
- 2.615E8
- 261500000
- 261.5E8
- 2.6
6. Select the correct example of the complex datatype in Python
- 3 + 2j
- -100j
- 5j
- All of the above are correct
7. What is the correct way of creating a multi-line string in Python?
- str = “”My name is Kevin and I
live in New York”” - str = “””My name is Kevin and I
live in New York””” - str = “My name is Kevin and I
live in New York” - All of the above
8. How to convert the uppercase letters in the string to lowercase in Python?
- lowercase()
- capitalize()
- lower()
- toLower()
9. How to access substring “Kevin” from the following string declaration in Python:
1 2 3 |
str = "My name is Kevin" |
- str[11:16]
- str(11:16)
- str[11][16]
- str[11-16]
10. Which of the following is the correct way to access a specific character? Let’s say we need to access the character “K” from the following string in Python.
1 2 3 |
str = "My name is Kevin" |
- str(11)
- str[11]
- str:9
- None of the above
11. Which Python module is used to parse dates in almost any string format?
- datetime module
- time module
- calendar module
- dateutil module
12. Which of the following is the correct way to indicate Hexadecimal Notation in Python?
- str = ‘\62’
- str = ’62’
- str = “62”
- str = ‘\x62’
13. To begin slicing from the end of the string, which of the following is used in Python?
- Indexing
- Negative Indexing
- Begin with the 0th index
- Escape Characters
14. How to fetch characters from a given range in Python?
- [:]
- in operator
- []
- None of the above
15. How to capitalize only the first letter of a sentence in Python?
- uppercase() method
- capitalize() method
- upper() method
- None of the above
16. What is the correct way to get the maximum value from Tuple in Python?
- print (max(mytuple));
- print (maximum(mytuple));
- print (mytuple.max());
- print (mytuple.maximum);
17. How to fetch and display only the keys of a Dictionary in Python?
- print(mystock.keys())
- print(mystock.key())
- print(keys(mystock))
- print(key(mystock))
18. How to align a string centrally in Python?
- align() method
- center() method
- fill() method
- None of the above
19. How to access value for key “Product” in the following Python Dictionary:
1 2 3 4 5 6 7 8 |
mystock = { "Product": "Earphone", "Price": 800, "Quantity": 50, "InStock" : "Yes" } |
- mystock[“Product”]
- mystock(“Product”)
- mystock[Product]
- mystock(Product)
20. How to set the tab size to 6 in Python Strings?
- expandtabs(6)
- tabs(6)
- expand(6)
- None of the above
21. ___________ uses square brackets for comma-separated values in Python? Fill in the blanks with a Python collection.
- Lists
- Dictionary
- Tuples
- None of the above
22. Are Python Tuples faster than Lists?
- TRUE
- FALSE
23. How to find the index of the first occurrence of a specific value “i”, from the string “This is my website”?
- str.find(“i”)
- str.find(i)
- str.index()
- str.index(“i”)
24. How to display whether the date is AM/PM in Python?
- Use the %H format code
- Use the %p format code
- Use the %y format code
- Use the %I format code
25. Which of the following is the correct way to access a specific element from a Multi-Dimensional List?
- list[row_size:column_size]
- list[row_size][column_size]
- list[(row_size)(column_size)]
- None of the above
26. Which of the following Bitwise operators in Python shifts the left operand value to the right, by the number of bits in the right operand? The rightmost bits while shifting fall off.
- Bitwise XOR Operator
- Bitwise Right Shift Operator
- Bitwise Left Shift Operator
- None of the Above
27. How to specify range of index in Python Tuples? Let’s say our tuple name is “mytuple”
- print(mytuple[1:4])
- print(mytuple[1 to 4])
- print(mytuple[1-4])
- print(mytuple[].slice[1:4])
28. How to correctly create a function in Python?
- demoFunction()
- def demoFunction()
- function demoFunction()
- void demoFunction()
29. Which one of the following is a valid identifier declaration in Python?
- str = “demo666”
- str = “666”
- str = “demo demo2”
- str = “$$$666”
30. How to check whether all the characters in a string is printable?
- print() method
- printable() method
- isprintable() method
- echo() method
31. How to delete an element in a Dictionary with a specific key. Let’s say we need to delete the key “Price”
- del dict[‘Price’]
- del.dict[‘Price’]
- del dict(‘Price’)
- del.dict[‘Price’]
32. How to swap cases in Python i.e. lowercase to uppercase and vice versa?
- casefold() method
- swapcase() method
- case() method
- title() method
33. The def keyword is used in Python to _______?
- Define a function name
- Define a list
- Define an array
- Define a Dictionary
34. Which of the following is used to empty the Dictionary in Python? Let’s say our dictionary name is “mystock”.
- mystock.del()
- clear mystock
- del mystock
- mystock.clear()
35. How to display only the day number of years in Python?
- date.strftime(“%j”)
- date.strftime(“%H”)
- date.strftime(“%I”)
- date.strftime(“%p”)
36. What is used in Python functions, if you have no idea about the number of arguments to be passed?
- Keyword Arguments
- Default Arguments
- Required Arguments
- Arbitrary Arguments
37. What is the correct way to create a Dictionary in Python?
- mystock = {“Product”: “Earphone”, “Price”: 800, “Quantity”: 50}
- mystock = {“Product”- “Earphone”, “Price”-800, “Quantity”-50}
- mystock = {Product[Earphone], Price[800], Quantity[50]}
- None of the above
38. In Python Functions, what is to be used to skip arguments or even pass them in any order while calling a function?
- Keyword arguments
- Default Arguments
- Required Arguments
- Arbitrary Arguments
39. How to get the total length of a Tuple in Python?
- count() method
- length() method
- len() method
- None of the above
40. How to access a value in Tuple?
- mytuple(1)
- mytuple{1}
- mytuple[1]
- None of the above
41. What is to be used to create a Dictionary of arguments in a Python function?
- Arbitrary arguments in Python (*args)
- Arbitrary keyword arguments in Python (**args)
- Keyword Arguments
- Default Arguments
42. How to convert the lowercase letters in the string to uppercase in Python?
- upper()
- uppercase()
- capitalize()
- toUpper()
43. What is the correct way to get the minimum value from a List in Python?
- print (minimum(mylist));
- print (min(mylist));
- print (mylist.min());
- print (mylist.minimum());
44. Is using a return statement optional in a Python Function?
- Yes
- No
45. Which of the following correctly convert int to float in Python?
- res = float(10)
- res = convertToFloat(10)
- None of the above
46. How to get the type of a variable in Python?
- print(typeOf(a))
- print(typeof(a))
- print(type(a))
- None of the above
47. How to compare two operands in Python and check for equality? Which operator is to be used?
- =
- in operator
- is operator
- == (Answer)
48. What is the correct way to get minimum value from Tuple?
- print (min(mytuple));
- print (minimum(mytuple));
- print (mytuple.min());
- print (mytuple.minimum);
49. How to display only the current month’s number in Python?
- date.strftime(“%H”)
- date.strftime(“%I”)
- date.strftime(“%p”)
- date.strftime(“%m”)
50. How to compare two objects and check whether they have the same memory locations?
- is operator
- in operator
- **
- Bitwise operators
51. How to fetch and display only the values of a Dictionary in Python?
- print(mystock.value())
- print(mystock.values())
- print(values(mystock))
- print(value(mystock))
52. Which operator is used in Python to raise numbers to the power?
- Bitwise Operators
- Exponentiation Operator (**)
- Identity Operator (is)
- Membership Operators (in)
53. Can we create a Tuple in Python without parenthesis?
- TRUE
- FALSE
54. ____________ represents key-value pair in Python?
- Tuples
- Lists
- Dictionary
- All the Above
55. Which of the following Bitwise operators sets each bit to 1, if only one of them is 1, i.e. if only one of the two operands is 1?
- Bitwise XOR
- Bitwise OR
- Bitwise AND
- Bitwise NOT
56. What is the correct way to get the maximum value from a List in Python?
- print (maximum(mylist));
- print (mylist.max());
- print (max(mylist));
- print (mylist.maximum());
57. An example to correctly begin searching from the end range of index in Python Tuples. Let’s say our Python Tuple has 4 elements
- print(mytuple[-3 to -1])
- print(mytuple[3-1])
- print(mytuple[].slice[-3:-1])
- print(mytuple[-3:-1])
58. Which of the following Bitwise operators in Python shifts the left operand value to the left, by the number of bits in the right operand? The leftmost bits while shifting fall off.
- Bitwise XOR Operator
- Bitwise Right Shift Operator
- Bitwise Left Shift Operator
- None of the Above
59. Can we update Tuples or any of its elements in Python after the assignment?
- Yes
- No
60. How to display only the current month’s name in Python?
- date.strftime(“%H”)
- date.strftime(“%B”)
- date.strftime(“%m”)
- date.strftime(“%d”)
61. ___________ uses Parenthesis for comma-separated values in Python? Fill in the blanks with a Python collection?
- List
- Tuples
- None of the above
62. How to create an empty Tuple in Python?
- mytuple = ()
- mytuple = (0)
- mytuple = 0
- mytuple =
63. Can we have duplicate keys in the Python Dictionary?
- TRUE
- FALSE
64. Lists in Python are Immutable?
- TRUE
- FALSE
65. What is the correct way to create a list with type float?
- mylist = [5.7, 8.2, 3.8, 2.9]
- mylist = (5.7, 8.2, 3.8, 2.9)
- mylist = {5.7, 8.2, 3.8, 2.9}
- None of the above
66. How to display only the day number of years in Python?
- date.strftime(“%j”)
- date.strftime(“%H”)
- date.strftime(“%I”)
- date.strftime(“%p”)
67. Delete multiple elements in a range from a Python List
- del mylist[2:4]
- del mylist(2:4)
- del mylist[2 to 4]
- del mylist(2 to 4)
68. How to fetch the last element from a Python List with negative indexing?
- print(“Last element = “,mylist[0])
- print(“Last element = “,mylist[])
- print(“Last element = “,mylist[-1])
- None of the above
69. What is the correct way to get the length of a List in Python?
- mylist.count()
- count(mylist)
- len(mylist)
- length(mylist)
70. To get today’s date, which Python module is to be imported?
- calendar module
- datetime module
- dateutil module
- None of the above
We saw the top 70 Multiple-choice Questions and Answers above. If you liked the tutorial, spread the word and share the link and our website Studyopedia with others.
For Videos, Join Our YouTube Channel: Join Now
Recommended Posts
- Java Interview Questions & Answers
- Android Interview Questions & Answers
- ReactJS Interview Questions & Answers
- C Programming Quiz
- C++ Quiz
- Android Quiz
- jQuery Quiz
- HTML5 Quiz
- CSS Quiz
- PHP Quiz
No Comments