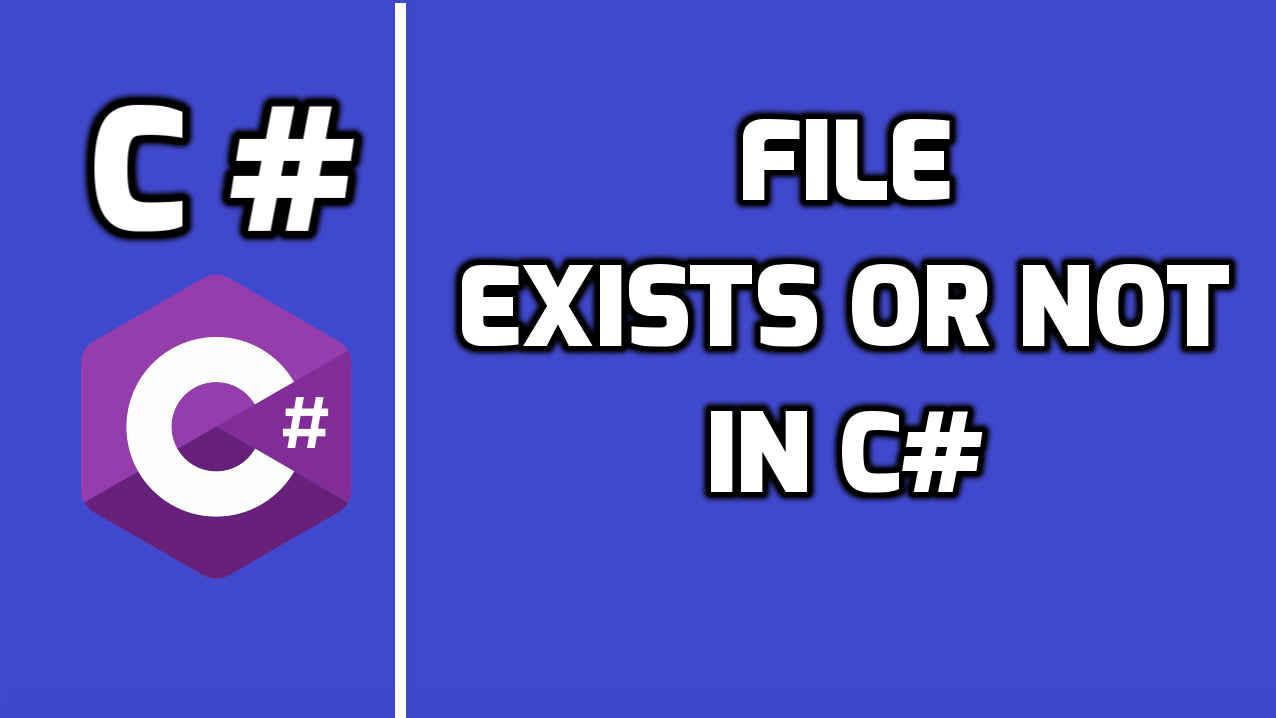
04 Oct C# Code to check if file exists or not
A simple C# program to check if a file exists. For this, use the File.Exists() method in C#. This allows us to check for both the current directory and a particular directory like C: D: E: etc.
- Current Directory
File.Exists(“new.txt”) - Particular Directory
File.Exists(@”E:\demo.txt”)
Let us see the code to check if the file exists in C#. Consider, we have a file “new.txt” in the current directory:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
using System; using System.IO; public class One { public static void Main() { // particular directory if (File.Exists(@"E:\demo.txt")) Console.WriteLine("File is in the directory"); else Console.WriteLine("File isn't in the directory"); // current directory if (File.Exists("new.txt")) Console.WriteLine("File is in the current directory"); else Console.WriteLine("File isn't in the current directory"); } } |
The output is as follows:
1 2 3 4 |
File isn't in the directory File is in the current directory |
Above, we have used the File.Exists() method to check for the existence of a file. The output displays that we have “new.txt” in the current directory.
To understand the code correctly, let us now see the following video:
No Comments