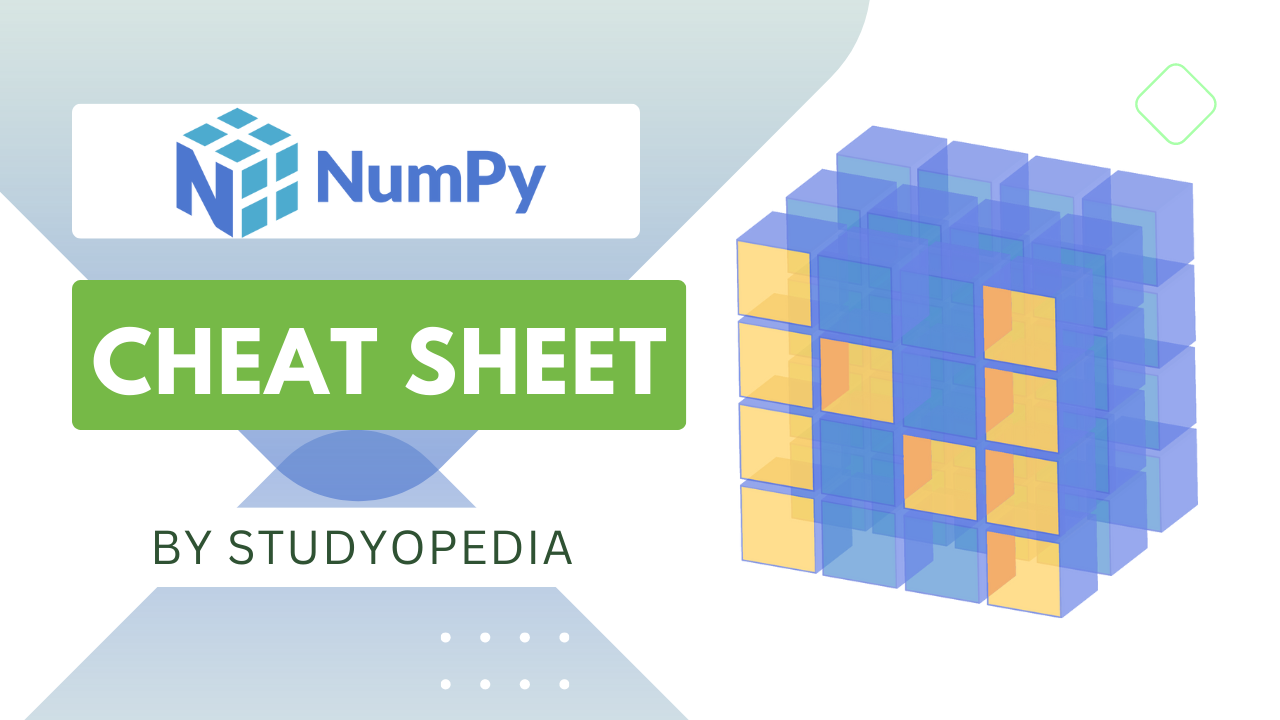
04 Aug NumPy Cheat Sheet
NumPy Cheat Sheet will guide you to work on NumPy with basics and advanced topics. Cheat Sheet for students, engineers, and professionals.
Introduction
NumPy is a Python library for numeric and scientific computing. It stands for numerical python and consists of multi-dimensional array objects.
Installation
To install NumPy, use the PIP package manager. Install Python and PIP, and then use PIP to install the NumPy Python library:
1 2 3 |
pip install numpy |
Create NumPy Arrays
To create Numpy arrays, useĀ numpy.arrays() method in Python:
- Create a Numpy array by passing a tuple:
1234n = np.array((1, 2, 3))print(n) - Create a Numpy array by passing a list:
1234n = np.array([1, 2, 3])print(n)
Dimensions of NumPy Arrays
Dimensions of an array in NumPy are also called the Rank of an Array. Check how many dimensions an array has used with theĀ numpy.ndarray.ndim:
- Create a Zero-dimensional NumPy array:
123456import numpy as npn = np.array(25)print("Dimensions = ",n.ndim) # Dimensions = 0 - Create a One-dimensional NumPy array:
123456import numpy as npn = np.array([10, 20, 30])print("Dimensions = ",n.ndim) # Dimensions = 1 - Create a Two-dimensional NumPy array:
123456import numpy as npn = np.array([[1,3,5],[4,8,12]])print("Dimensions = ",n.ndim) # Dimensions = 2
Initialize NumPy Arrays
To Initialize NumPy arrays, use the numpy.zeros() method. Let us see how to initialize numpy arrays with zeros:
1 2 3 4 5 6 |
import numpy as np n = np.zeros([2, 3]) print(n) |
Output
1 2 3 4 |
[[0. 0. 0.] [0. 0. 0.]] |
Array Indexing
Array indexing is accessing array elements. In Numpy, access an array element using the index number. The 0th index is element 1 and the flow goes on as shown below:
- Ā index 0 ā element 1
- Ā index 1 ā element 2
- Ā index 2 ā element 3
- Ā index 3 ā element 4
Let us see some examples:
- Access the 1st element (index 0) from a One-Dimensional array:
123456import numpy as npn = np.array([10, 20, 30, 40, 50])print(n[0]) # 10 - Accessing 1st dimension elements from a 2D array:
1234567import numpy as npn = np.array([[1,3,5],[4,8,12]])print(n[0,1]) # 3 - Access the last element from a 1D array with negative indexing:
123456import numpy as npn = np.array([5, 10, 15])print(n[-1]) # 15
Array Slicing
Slicing the array in a range from start to end is what we call Array Slicing. The following is the syntax to slice NumPy arrays. Here,Ā startĀ is the beginning index (include), and theĀ end is the last index (excluded):
1 2 3 |
arr[start:end} |
Let us see some examples:
- Slicing from index 1 to 3 (3 is excluded):
123456import numpy as npn = np.array([20, 25, 30, 35, 40, 45, 50])print(n[1:3]) # [25 30] - Slicing from index 5 to last:
123456import numpy as npn = np.array([20, 25, 30, 35, 40, 45, 50])print(n[:5]) # [20 25 30 35 40]
NumPy Datatypes
Python Numpy supports the following data types:
- b ā boolean
- u ā unsigned integer
- f ā float
- c ā complex float
- m ā timedelta
- M ā datetime
- O ā object
- S ā string
- U ā unicode string
Let us see some examples:
- Get the datatype of a Numpy array with strings:
123456import numpy as npn = np.array(["amit", "rohit", "ashwin", "jadeja", "virat"])print(n.dtype) # <U6 - Convert string to integer:
1234567891011import numpy as npn = np.array(['10', '20', '30', '40', '50'])print(n)print(n.dtype)n2 = n.astype('i')print(n2)print(n2.dtype)
Output
123456['10' '20' '30' '40' '50']<U2[10 20 30 40 50]int32
NumPy Array Shape
In Python Numpy, the number of elements in each dimension of an array is called the shape. To get the shape of a Numpy array, use theĀ numpy.shape attribute in Python:
- Check the shape of a Zero-Dimensional NumPy array:
123456import numpy as npn = np.array(10)print(n.shape) # () - Check the shape of a One-Dimensional NumPy array:
123456import numpy as npn = np.array([10, 20, 30])print(n.shape) # (3,)
Reshape a NumPy Array
To reshape the number of dimensions, use theĀ numpy.reshape() method in Python. Reshape allows you to edit the number of elements in a dimension. Let us reshape dimensions from 1D to 2D:
1 2 3 4 5 6 7 8 9 10 11 12 |
import numpy as np # Create a Numpy array n = np.array([5, 10, 15, 20, 25, 30]) print("One-Dimensional Array = ",n) # Reshape the array resarr = n.reshape(2, 3) print("Reshaped array (Two-Dimensional) = \n",resarr) |
Output
1 2 3 4 5 6 |
One-Dimensional Array = [ 5 10 15 20 25 30] Reshaped array (Two-Dimensional) = [[ 5 10 15] [20 25 30]] |
Iterate Numpy Arrays
Iteration displays each element of an array. We will see how to iterate through Numpy arrays using loops. Let us iterate a One-Dimensional Numpy array:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
import numpy as np # Create a Numpy 1D array n = np.array([5, 10, 15, 20, 25, 30]) print("One-Dimensional Array = ",n) print("Type = ",type(n)) print("DataType = ",n.dtype) print("\nIterating...") for a in n: print(a) |
Output
1 2 3 4 5 6 7 8 9 10 11 12 13 |
One-Dimensional Array = [ 5 10 15 20 25 30] Type = <class 'numpy.ndarray'> DataType = int32 Iterating... 5 10 15 20 25 30 |
Joining NumPy Arrays
We can join two or more arrays in a single new array:
1 2 3 4 5 6 7 8 9 10 11 |
import numpy as np # Create two arrays n1 = np.array([5, 10, 15, 20]) n2 = np.array([25, 30, 35, 40]) # Joining arrays resarr = np.concatenate((n1, n2)) print("\nAfter Joining = ", resarr) |
Output
1 2 3 |
After Joining = [ 5 10 15 20 25 30 35 40] |
Join arrays in Numpy using the stack methods, such as:
- stack(): Join arrays
- hstack(): Stack along rows
- vstack(): Stack along columns
- dstack(): Stack along depth
- column_stack(): Stack according to columns
Split NumPy Array
To split an array, use the array_split()Ā method. Following is the syntax:
1 2 3 |
array_split(arr_name, split_num) |
Here,Ā arr_nameĀ is the name of the array,Ā split_numĀ is the count of splits.
Let us see how to split a 1d array:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
import numpy as np # Create a Numpy array n = np.array([10, 20, 30, 40, 50, 60]) # splitting array into 2 resarr = np.array_split(n, 2) print("\nArray after splitting i.e. returns 2 arrays"); for a in resarr: print(a) |
Output
1 2 3 4 5 |
Array after splitting i.e. returns 2 arrays [10 20 30] [40 50 60] |
Search an array for a value
In a Numpy array, we can search for a specific value using theĀ numpy.where() method. This method returns the index where the specific element is found. Let us see an example:
1 2 3 4 5 6 7 8 9 10 11 12 |
import numpy as np # Create a Numpy Array n = np.array([10, 20, 30, 40, 50, 30]) # Searching a specific value 30 res = np.where(n == 30) print("\nElement 30 found at following indexes:") print(res) |
Output
1 2 3 4 |
Element 30 found at following indexes: (array([2, 5], dtype=int64),) |
Sort Arrays
TheĀ numpy.sort() function is used in Numpy to sort arrays in a sequence. This sequence can be ascending or descending. Let us see how to sort arrays:
1 2 3 4 5 6 7 8 9 |
import numpy as np # Create a 1D integer array n = np.array([50, 25, 35, 15, 55, 75, 65, 60, 30, 45]) # Sort the array using the sort() method print("\nSorted array = ", np.sort(n)) |
Output
1 2 3 |
Sorted array = [15 25 30 35 45 50 55 60 65 75] |
Axes in Arrays
In Numpy arrays, the axis is the direction along the rows and columns. A two-dimensional numpy array has the following two corresponding axes:
1 2 3 4 |
vertical axis (axis 0) horizontal axis (axis 1) |
Let us see an example to get the minimum value with axes:
1 2 3 4 5 6 7 8 9 10 |
import numpy as np # Create a numpy 3x3 array n = np.array([[2, 5, 7, 9, 6], [15, 25, 35, 45, 55], [100, 200, 300, 400, 500]]) print("\nMinimum value = ", n.min()) print("\nMinimum value with axis 0 (vertical axes) = ", n.min(axis=0)) print("\nMinimum value with axis 1 (horizontal axes) = ", n.min(axis=1)) |
Output
1 2 3 4 5 |
Minimum value = 2 Minimum value with axis 0 (vertical axes) = [2 5 7 9 6] Minimum value with axis 1 (horizontal axes) = [ 2 15 100] |
Intersection of NumPy Arrays
If you want to get the intersection of two arrays, use theĀ intersect1d() method in Numpy. Intersection means finding common elements between two arrays.Ā Let us see an example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
import numpy as np # Create two arrays n1 = np.array([5, 10, 15, 20]) n2 = np.array([5, 15, 30, 40]) print("Iterating array1...") for a in n1: print(a) print("\nIterating array2...") for a in n2: print(a) # Find intersection using the intersect1d() method resarr = np.intersect1d(n1, n2) print("\nIntersection = \n", resarr) |
Output
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
Iterating array1... 5 10 15 20 Iterating array2... 5 15 30 40 Intersection = [ 5 15] |
Difference between NumPy Arrays
To find the difference between two arrays, use theĀ numpy.setdiff1d() method. This means if we are subtracting two arrays, then setdiff1d() will subtract the common elements from the 1st array and display the rest of the elements of the 1st array. Let us see an example:
1 2 3 4 5 6 7 8 9 10 11 |
import numpy as np # Create two arrays n1 = np.array([5, 10, 15, 20, 25, 30, 35, 40, 45, 50]) n2 = np.array([30, 35, 50, 55, 60, 65, 75, 85, 95, 100]) # n1 - n2 diffarr = np.setdiff1d(n1, n2) print("\nDifference = \n", diffarr) |
Output
1 2 3 4 |
Difference = [ 5 10 15 20 25 40 45] |
Arithmetic operations between NumPy Arrays
Perform arithmetic operations on Numpy arrays:
- Add Numpy Arrays: Use theĀ sum() method to add arrays:
1234567891011import numpy as np# Create arraysn1 = np.array([15, 20, 25, 30])n2 = np.array([65, 75, 85, 95])# Add the arrays elementsresarr = np.sum([n1, n2])print("Sum = ", resarr) # Sum = 410 - Subtract Numpy Arrays: Use theĀ subtract() method to subtract one array from another:
1234567891011import numpy as np# Create two arraysn1 = np.array([15, 20, 25, 30])n2 = np.array([65, 75, 85, 95])# Subtract Numpy arraysresarr = np.subtract(n1, n2)print("Subtraction result = ", resarr) # [-50 -55 -60 65] - Multiply Numpy Arrays: Use theĀ multiply() method to multiply elements of one array to another:
1234567891011import numpy as np# Create Numpy arraysn1 = np.array([15, 20, 25, 30])n2 = np.array([65, 75, 85, 95])# Multiply Numpy arraysresarr = np.multiply(n1, n2)print("Multiplication result = ", resarr) # [975 1500 2125 2850] - Divide Numpy Arrays: TheĀ divide() method divides elements of one array with elements of another:
1234567891011import numpy as np# Create two Numpy arraysn1 = np.array([15, 20, 25, 30])n2 = np.array([65, 75, 85, 95])# Divide arraysresarr = np.divide(n2, n1)print("Division Result = ", resarr) # [4.33333333 3.75 3.4 3.16666667]
Scalar Operations on NumPy Arrays
Scalar operations on Numpy arrays include performing:
- Addition Operation on Numpy Arrays: The Addition Operation adds a value to each element of a NumPy array:
123456n = np.array([15, 20, 25, 30])# performing addition on each elementn = n + 10; - Subtraction Operation on Numpy Arrays: The Subtraction operation is subtracting a value from each element of a NumPy array:
123456n = np.array([15, 20, 25, 30])# performing subtraction on each elementn = n - 10; - Multiplication Operation on Numpy Arrays: The Multiplication operation is multiplying a value to each element of a NumPy array:
123456n = np.array([15, 20, 25, 30])# performing multpilication on each elementn = n * 10; - Division Operation on Numpy Arrays: The Division operation is to divide a value from each element of a NumPy array:
123456n = np.array([15, 20, 25, 30])# performing division on each elementn = n / 5;
Statistical Operations on NumPy Arrays
We can perform statistical operations, like mean, median, and standard deviation on NumPy arrays:
- Mean: Mean is the average of the given values. Here, we will find the mean of the array elements using theĀ mean() method:
1234567891011import numpy as np# Create two arraysn = np.array([15, 20, 25, 30])# meanresmean = np.mean(n);print("\nMean = ", resmean) # 22.5 - Median: Median is the middle value of the given values. Here, we will find the median of the array elements using theĀ median() method:
1234567891011import numpy as np# Create two arraysn = np.array([15, 20, 25, 30])# medianresmedian = np.median(n);print("\nMedian = ", resmedian) # 22.5 - Standard Deviation: Standard Deviation is a measure of the amount of variation or dispersion of the given values. Here, we will find the standard deviation of the array elements using theĀ std() method:
1234567891011import numpy as np# Create two arraysn = np.array([15, 20, 25, 30])# standard deviationresmean = np.mean(n);print("\nStandard Deviation = ", resmean) # 5.5901699437494745
What’s next?
If you liked the tutorial, spread the word and share the link and our website Studyopedia with others.
For Videos, Join Our YouTube Channel: Join Now
No Comments